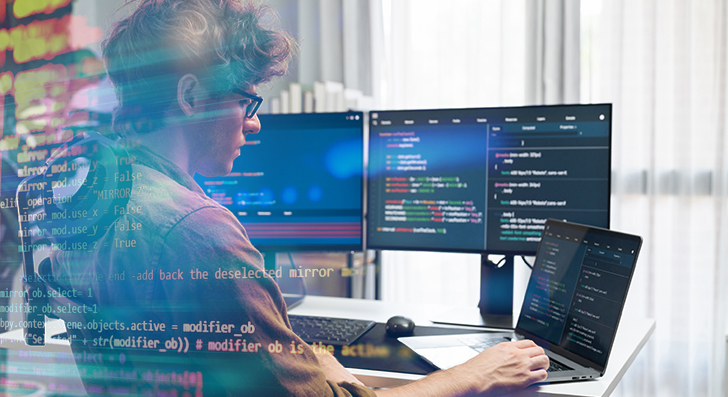
Scalability usually means your application can deal with growth—extra people, far more information, and much more traffic—without breaking. For a developer, creating with scalability in mind will save time and tension afterwards. Here’s a transparent and useful guide to assist you to start off by Gustavo Woltmann.
Layout for Scalability from the Start
Scalability just isn't some thing you bolt on later—it ought to be portion of one's approach from the start. Many apps fail if they increase rapidly since the original style and design can’t deal with the additional load. As being a developer, you'll want to Feel early regarding how your process will behave under pressure.
Start by building your architecture to be adaptable. Keep away from monolithic codebases exactly where everything is tightly linked. Alternatively, use modular style or microservices. These styles break your application into smaller sized, unbiased parts. Just about every module or services can scale on its own devoid of affecting The entire method.
Also, give thought to your database from day just one. Will it need to deal with one million customers or maybe 100? Choose the appropriate kind—relational or NoSQL—according to how your facts will grow. System for sharding, indexing, and backups early, even if you don’t want them nonetheless.
An additional critical place is to avoid hardcoding assumptions. Don’t compose code that only is effective beneath present-day conditions. Take into consideration what would come about if your user base doubled tomorrow. Would your app crash? Would the databases decelerate?
Use layout designs that assist scaling, like concept queues or function-driven techniques. These aid your app deal with much more requests with out finding overloaded.
Any time you Make with scalability in your mind, you're not just getting ready for success—you're reducing future problems. A nicely-prepared procedure is less complicated to keep up, adapt, and develop. It’s much better to prepare early than to rebuild afterwards.
Use the best Database
Deciding on the right databases can be a essential Portion of building scalable purposes. Not all databases are created the identical, and using the Completely wrong you can sluggish you down and even cause failures as your application grows.
Commence by comprehending your details. Could it be extremely structured, like rows inside of a table? If Indeed, a relational database like PostgreSQL or MySQL is a great suit. They are solid with associations, transactions, and regularity. Additionally they support scaling tactics like study replicas, indexing, and partitioning to handle far more visitors and facts.
Should your details is more adaptable—like user action logs, product catalogs, or paperwork—think about a NoSQL solution like MongoDB, Cassandra, or DynamoDB. NoSQL databases are much better at dealing with large volumes of unstructured or semi-structured knowledge and will scale horizontally much more quickly.
Also, think about your examine and write styles. Are you currently undertaking lots of reads with fewer writes? Use caching and browse replicas. Are you presently handling a weighty generate load? Consider databases that could deal with substantial produce throughput, or even occasion-based mostly facts storage systems like Apache Kafka (for short-term knowledge streams).
It’s also good to think ahead. You might not have to have advanced scaling attributes now, but selecting a database that supports them signifies you received’t have to have to modify afterwards.
Use indexing to hurry up queries. Avoid unneeded joins. Normalize or denormalize your facts based upon your obtain styles. And always monitor database performance as you grow.
In short, the proper databases is dependent upon your app’s construction, speed requirements, and how you anticipate it to grow. Take time to select sensibly—it’ll help save lots of trouble afterwards.
Improve Code and Queries
Rapidly code is vital to scalability. As your app grows, every compact hold off adds up. Badly written code or unoptimized queries can slow down overall performance and overload your system. That’s why it’s imperative that you Establish successful logic from the start.
Begin by writing clean up, basic code. Stay away from repeating logic and remove just about anything pointless. Don’t pick the most advanced Remedy if a simple just one operates. Keep your features brief, concentrated, and simple to test. Use profiling instruments to search out bottlenecks—areas where your code can take also long to run or uses an excessive amount memory.
Up coming, look at your database queries. These often sluggish items down much more than the code by itself. Be certain Every single question only asks for the data you truly require. Prevent Choose *, which fetches anything, and rather pick out distinct fields. Use indexes to hurry up lookups. And stay away from executing too many joins, Specifically throughout big tables.
When you notice the exact same information staying asked for time and again, use caching. Store the outcome quickly using resources like Redis or Memcached therefore you don’t have to repeat pricey functions.
Also, batch your databases functions whenever you can. In place of updating a row one after the other, update them in groups. This cuts down on overhead and can make your application extra efficient.
Remember to check with massive datasets. Code and queries that do the job fine with 100 records may well crash whenever they have to manage 1 million.
In brief, scalable apps are rapidly applications. Maintain your code restricted, your queries lean, and use caching when essential. These techniques assistance your software continue to be sleek and responsive, at the same time as the load improves.
Leverage Load Balancing and Caching
As your application grows, it has to deal with a lot more consumers and even more targeted traffic. If anything goes by just one server, it can promptly turn into a bottleneck. That’s the place load balancing and caching can be click here found in. These two resources support maintain your app fast, stable, and scalable.
Load balancing spreads incoming targeted visitors throughout a number of servers. As an alternative to a single server carrying out all of the work, the load balancer routes users to distinctive servers based upon availability. This suggests no solitary server gets overloaded. If one server goes down, the load balancer can mail visitors to the Other people. Tools like Nginx, HAProxy, or cloud-centered remedies from AWS and Google Cloud make this simple to set up.
Caching is about storing facts briefly so it can be reused immediately. When buyers ask for exactly the same information yet again—like a product page or simply a profile—you don’t ought to fetch it in the databases whenever. You are able to provide it in the cache.
There's two frequent varieties of caching:
one. Server-aspect caching (like Redis or Memcached) suppliers knowledge in memory for fast entry.
2. Customer-side caching (like browser caching or CDN caching) merchants static files near to the person.
Caching lowers databases load, enhances velocity, and can make your application a lot more economical.
Use caching for things that don’t improve usually. And normally ensure your cache is current when information does transform.
In short, load balancing and caching are basic but powerful resources. Alongside one another, they assist your application deal with far more users, remain rapid, and recover from difficulties. If you propose to grow, you may need both of those.
Use Cloud and Container Resources
To develop scalable applications, you may need applications that permit your app increase easily. That’s in which cloud platforms and containers are available. They give you versatility, lower setup time, and make scaling Significantly smoother.
Cloud platforms like Amazon World-wide-web Services (AWS), Google Cloud Platform (GCP), and Microsoft Azure Allow you to lease servers and companies as you would like them. You don’t should invest in hardware or guess long term ability. When targeted traffic boosts, you could add more resources with just a few clicks or immediately making use of automobile-scaling. When site visitors drops, you'll be able to scale down to save cash.
These platforms also supply providers like managed databases, storage, load balancing, and stability applications. You could deal with making your application as an alternative to controlling infrastructure.
Containers are Yet another important tool. A container packages your application and anything it should run—code, libraries, settings—into a person device. This causes it to be uncomplicated to move your app concerning environments, from the laptop computer towards the cloud, without surprises. Docker is the preferred Resource for this.
When your application works by using several containers, resources like Kubernetes help you regulate them. Kubernetes handles deployment, scaling, and recovery. If 1 section of the application crashes, it restarts it immediately.
Containers also enable it to be very easy to separate aspects of your app into services. You may update or scale elements independently, which is great for performance and trustworthiness.
In brief, applying cloud and container equipment usually means it is possible to scale fast, deploy simply, and Recuperate quickly when troubles occur. In order for you your app to increase without boundaries, get started making use of these applications early. They conserve time, lower risk, and allow you to continue to be focused on creating, not correcting.
Keep track of Almost everything
If you don’t observe your application, you gained’t know when points go wrong. Monitoring will help the thing is how your application is performing, place troubles early, and make improved decisions as your app grows. It’s a essential Element of building scalable techniques.
Start off by monitoring essential metrics like CPU usage, memory, disk Area, and response time. These show you how your servers and solutions are carrying out. Equipment like Prometheus, Grafana, Datadog, or New Relic may help you obtain and visualize this data.
Don’t just check your servers—keep an eye on your application way too. Control how much time it's going to take for buyers to load internet pages, how frequently faults happen, and where they occur. Logging tools like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly can help you see what’s taking place inside your code.
Create alerts for critical difficulties. By way of example, When your response time goes over a limit or a service goes down, you should get notified immediately. This allows you take care of difficulties rapidly, typically just before consumers even discover.
Monitoring is also practical any time you make alterations. Should you deploy a brand new characteristic and see a spike in glitches or slowdowns, it is possible to roll it back right before it will cause true harm.
As your application grows, targeted traffic and information increase. Devoid of monitoring, you’ll miss indications of difficulty until it’s way too late. But with the proper applications in position, you stay on top of things.
In short, checking assists you keep the application dependable and scalable. It’s not pretty much spotting failures—it’s about being familiar with your program and ensuring that it works perfectly, even under pressure.
Closing Ideas
Scalability isn’t only for large corporations. Even little applications need a robust Basis. By developing diligently, optimizing properly, and utilizing the right equipment, you can Construct applications that develop efficiently without breaking stressed. Begin modest, think huge, and Make smart.